FORTH
I’ve started implementing my own FORTH language and posting some screen shots online. I won’t go into all the reasons why I’m doing this apart from saying I miss using FORTH. Now, I’m enjoying “rolling my own”.
Several people have asked if I’ve posted the code. The short answer is no. First, this is more of a hobby project to see if I can do it and how difficult it is to do. Second, being a hobby project, there are no tests, no elegant answers, just code that works. With bugs. It’s a prototype. Will I ever post the code? Maybe. I’m thinking about it.
So, what am I really doing? I’m implementing FORTH in Python. Why Python? It’s fast, it’s iterable, and I don’t need to worry about a tool chain that takes half an hour to “prepare devices”. That means I can code on the iPad, which is what I’m using for this. The code runs remotely on a RaspberryPi, but there isn’t anything to keep it from running on other platforms. I’ve run it on my MacBookPro without problem. That’s why Python is nice. But isn’t Python slow? I don’t know what you’re trying to execute, but anything that runs within half a second is fast enough for most things. I’ll worry if something takes more than 5 seconds. After all, I wait for XCode for ten minutes at times.
Right now, I’ve written about two-thirds of the words for a 79-standard FORTH. Some of these are now actually written in FORTH. So this proof-of-concept works. Here’s the thing. It’s implemented to work, not be efficient, or even based on anything done previously. It’s messy. The most difficult part is memory. FORTH uses raw memory for most things, and most FORTHs are written in assembler. Python, as most languages don’t allow hardware memory access. No, I’m not going to write assembler or ‘c’ calls to assembler. I’m not getting paid to do this. It’s fun for me.
As a side note, I can also create a GUI, call the underlying OS, and even control the GPIO pins on the RaspberryPi. That’s the beauty of Python as an implementation language.
If I can’t use memory, what am I doing? Well, initially, I used a standard Python list (array) of 32K. The problem is it’s not a byte-array. It’s just a list, so each element can hold anything pretty much. It works. It’s inefficient, but it’s simple to program.
I’m ripping out the generic list and rewriting my app to use an array of bytes. Now, Python has a bytearray object, but it’s messy. So, what I’m doing is putting constraints on a Python list to only hold a byte per element. Again, nothing fancy, but simple and it works. The big problem is, now I need to rework most of my logic to deal with this new simulated memory (SM) structure. I need to create low-level memory access routines before I can deal with using them in FORTH. I’m also writing my own memory dump routine. Along the way, I’ll start adding test code.
Two steps forward. One step back.
I also think I need to figure out (read learn) how to use Sphinx to generate documentation.
So, I could post the code, but it’s in a lot of flux at this point. No, I won’t use GitHub. If I post it, it would probably just either a zip file or source listings.
It’s a fun side project. I’m learning a lot about what FORTH does under-the-covers.
WWDC Hopes and Dreams Shattered?
What do I want from this year’s WWDC? Everything I’ve wanted over the past 4-5 WWDCs. Additionally, I would like (not want) more APIs for existing Apple apps, such as Journal, Books, etc. Also, a new Time Machine product. Support for more programming languages within XCode: Python, Ruby, Erlang;—at least Lua. Everyone supports Lua, right?
I’m not interested in VisionPro and its APIs until normal humans can afford the device.
I doubt Apple will ever create a visual programming layer over Swift/SwiftUI. That’s now obvious. Someone still could and should. I would if I had time and a fraction of the resources Apple has.
Apple doesn’t care about gaming, beyond what it brings in money-wise.
I miss Steve. I miss Steve’s pragmatism. I miss Steve’s “…one more thing.”
I’m hoping Apple’s next big thing will be a “super”-Oura ring. They have the tech. I love the Oura ring. It’s given me more insights into my sleep than anything Apple or third-party sleep app has.
Apple buys Nintendo? That would be a perfect match, but it will never happen. Also, as I said, Apple hates games. The best I can hope for is an Apple/Nintendo hardware collaboration, but that will not happen.
What if Apple had backward compatibility going back to System 7.0? That would be killer (for some).
What could you do if Apple devices had proximity sensors? Or temperature, pressure, light, sound sensors built-in, with appropriate APIs?
I want Apple to support NoSQL. MongoDB, CouchDB, etc. At least provide APIs to those products. Apple seems to assume relational is better. Wrappers are easy to create. Apple should.
When was the last time Apple updated the FileWrapper API?
I still think getting rid of the data and resource forks was a mistake. Now, everything needs to be compiled-in.
Swift and SwiftUI are great. They are, however, obtuse syntax-wise and crap (a scientific term) at prototyping. Apple needs a good prototyping language. Easy to learn/get into, and useful.
If I ruled the world, things would be different. Not better, just different.
Auteureist Reborn
Back in the good old days of skeuomorphism, the days of the first iPhone, I created a writing app for iOS. Scrivener™ had recently come out on the Mac, and I wanted something similar for the iPhone because I wanted to write on the new cool gadget.
It was a weird idea then, and still is. Who would want to write a short story, much less a full novel, on such a small device? I would. So, I created Auteureist. It was written in ObjectiveC and used old-style XIBs instead of Storyboards to lay out the UI. I pushed it out to the App Store, and it garnered a bit of a following, but never really took off.
That’s fine.
I wrote it for myself and designed it the way I work and write, incorporating some ideas from Scrivener. In fact, later versions could import and export Scrivener projects. I used Auteureist on the iPhone to write a full novel using the phone’s keyboard and my thumbs. I never published that novel.
Anyway, time passed, and the iPhone spawned the iPad. I reworked the app to run on the first iPads. Then ObjectiveC gave way to Swift. I redesigned and reworked the app to use the new-fangled, so-called, easy-to-learn language. My code was buggy and inefficient since I was learning a brand new language. I never pushed that app to the store.
Then storyboards and XIBs gave rise to SwiftUI. Well, not really, but you get the point. I did another redesign using SwiftUI. It held promise, but the code was messy and fraught with bugs. Swift, SwiftUI, and my knowledge of them grew with time and recently, I decided I didn’t like the old code, so I tossed it into my archives and began anew.
I now have what I believe is the best version of Auteureist ever. The code is streamlined, more stable, and efficient. Today, I felt it was good enough to transition my new writing to the new version of Auteureist, from the old ObjectiveC/Swift version.
The app is far from done, but the core features are there and work. It’s stable, has a smaller footprint, and the way I store writing projects is more compact and better organized. I’ve learned a lot both in Swift, SwiftUI and how I now write.
Regardless of how good I think the app is, I don’t think I’ll ever release it. First, it’s a highly opinionated app that works the way I work and want it to. Second, I don’t want to deal with supporting the app beyond my own needs.
I still want a better icon.
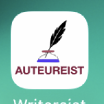
Generating Forms in SwiftUI - Part 3
This is just a quick post to show a concrete example of what I can do using my form-generation view.
Here is a screenshot of part of my app, with a form that was dynamically generated (not hard-coded in SwiftUI) in the inspector.
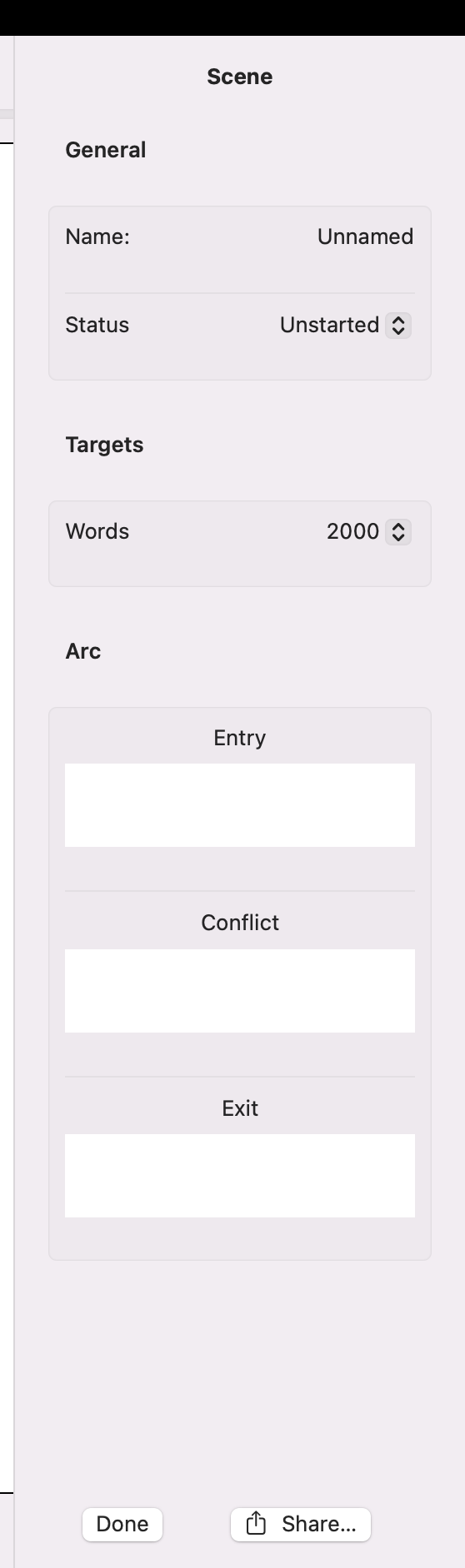
And here is the JSON that defines the form:
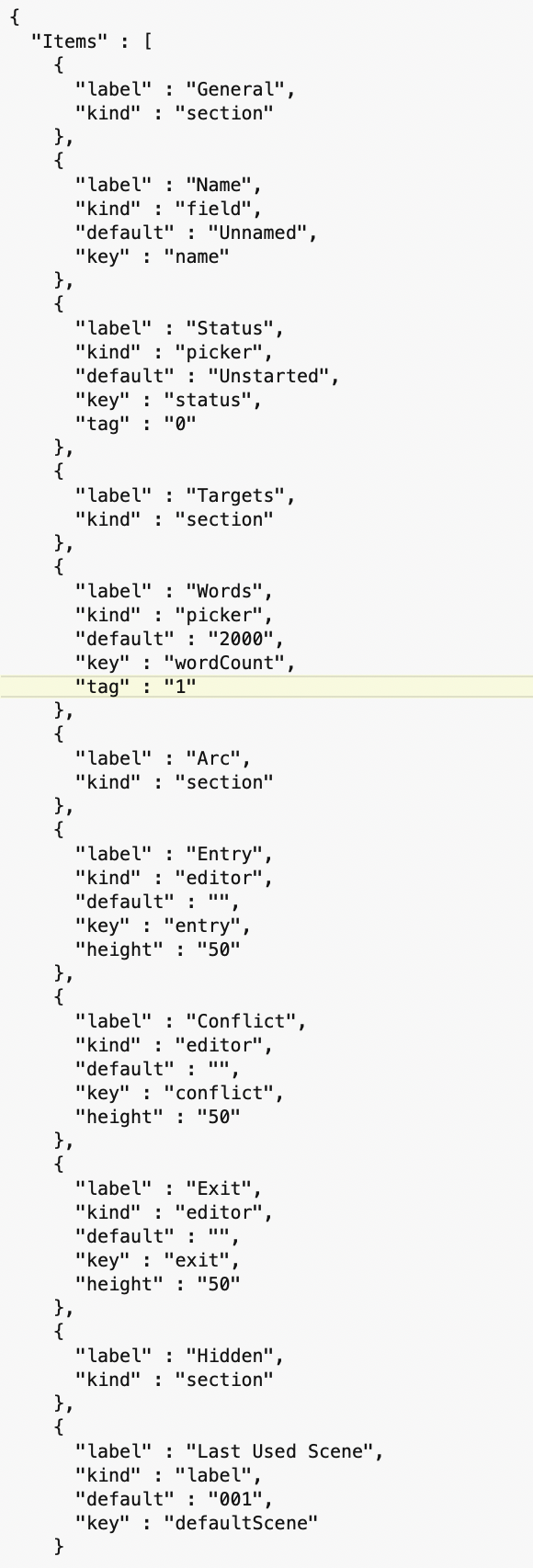
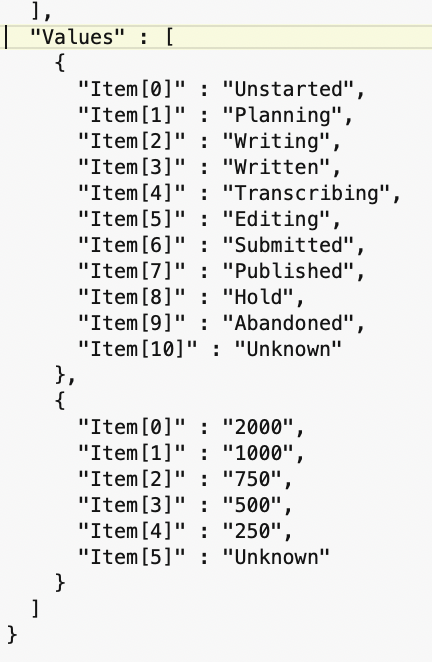
Generating Forms in SwiftUI - Part 2
In the previous post I mentioned I’ve been using a method for generating SwiftUI forms dynamically. In this post I’ll explain how I do so.
My forms are defined in JSON and stored in my project’s FileWrapper. That is,the forms are not defined in SwiftUI in the Xcode project. This lets me decouple the presentation of the form from the definition of the form so that if I decide to change the data or the form, my core app will still be able to function.
Here is an example of my JSON form metadata, for a single form. This is metadata since it doesn't have the actual values to be displayed, only defaults.
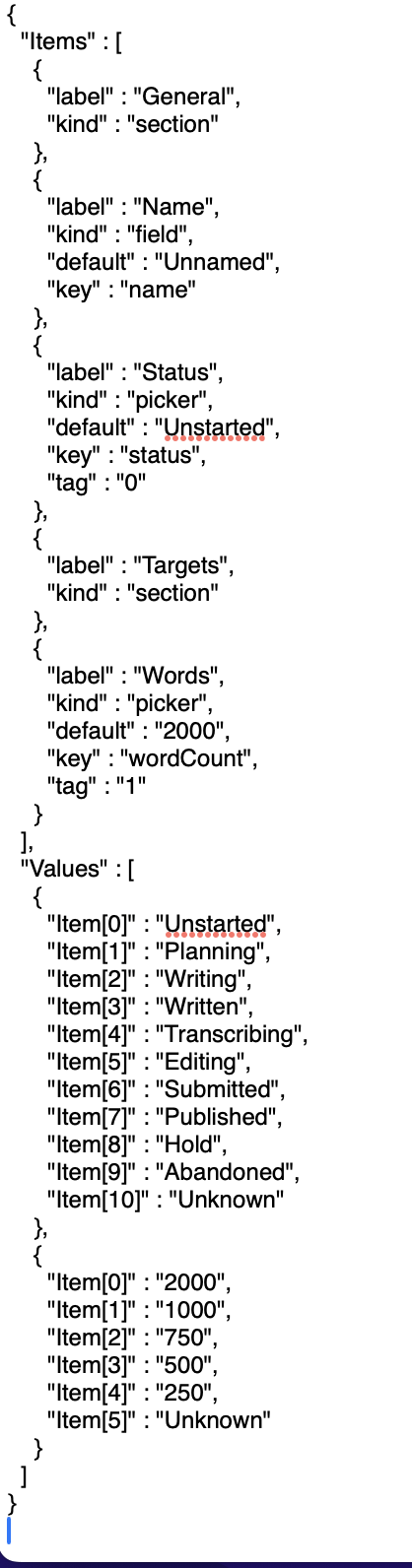
The JSON file has two top level entities: Items, and Values. Items are required, Values, are optional depending on the item.
Each item in Items defines a SwiftUI widget to be displayed on the form, and the items are in the order of display, top to bottom.
Each item consists of the following attributes:
- "label" : The label on the form for the widget,
- "kind" : The name of the widget to display,
- "default" : A default value to be displayed
- "key" : The key in the data model to which this widget maps
- "tag" : Optional. If kind is “picker”, this is the index of the Values item which contains an array of values for the picker.
I’ve made three simplifying assumptions when creating this structure:
- The form has no nesting, it’s a flat structure.
- All values returned and displayed by the form are of type String.
- I use string names for widgets to make it readable and to allow me to create my own widgets that are composites of others.
In order to display the form, I read the JSON from my FileWrapper, decode it into Swift array of type [[[String:String]]]. This is then passed to my SwiftUI view that generates the actual form. Here is a simplified example of the form generator view.
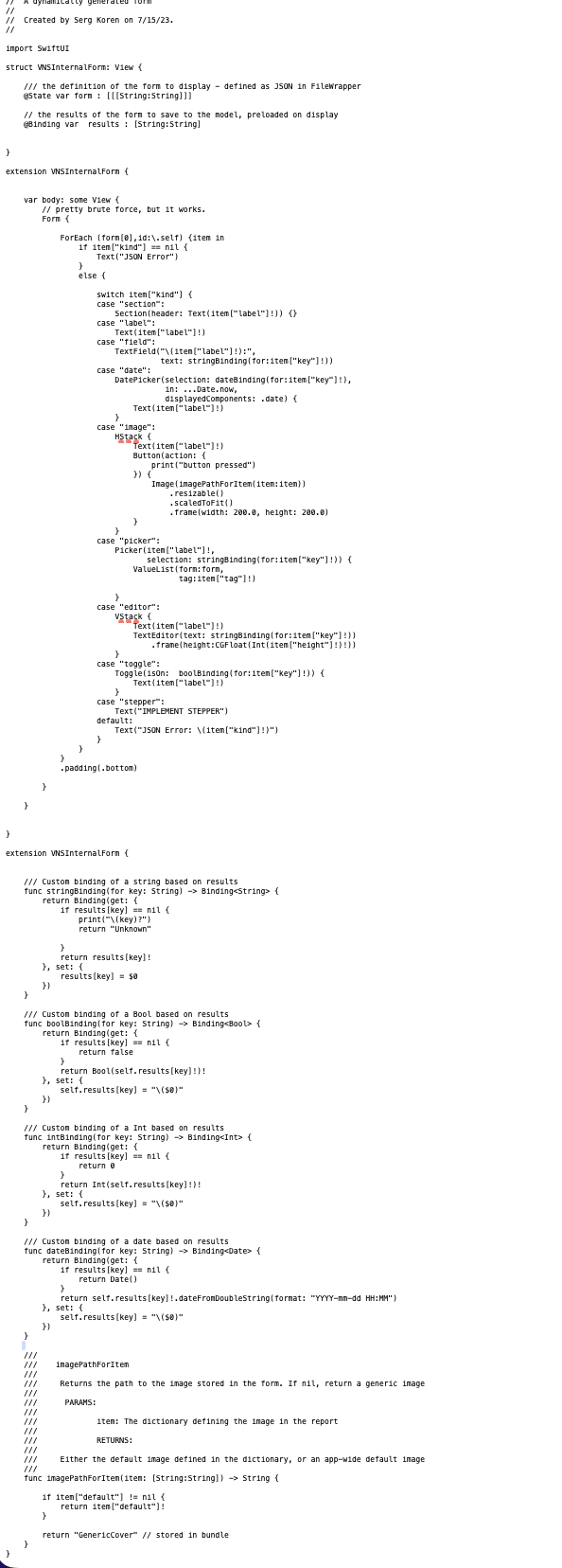
This form can be expanded with additional widgets, and the code could be simplified, but I’m showing you an example I hope is understandable. I’ve omitted a lot of error checking and some other widgets I have.
The results dictionary is preloaded (outside this view) with the current model values. On exit from this view, results has the data entered from the form, and is used to load back into the model.
That’s it. It may not be an optimal solution, but it works for what I need.
Generating Forms in SwiftUI - Part 1
One thing I came up with in the days of ObjectiveC, XIBs, and storyboards (remember those?) was a way to dynamically generate and handle forms. This method has evolved and streamlined, through all the Apple releases and I’ve finally come upon a simple way of doing it in Swift and SwiftUI.
Why would you want to generate a form? In my writing app, Auteureist, I have a ton of user-managed metadata. This is split across many forms. For example: a project metadata form, a form for character, locations, preferences, notes, research, statistics, etc. I didn’t want to lay out each form by hand in SwiftUI. Yes, it’s a lot easier than it used to be in the days of ObjectiveC, but it’s still repetitive. And what happens if I need to change a data structure that’s tied to a form? I’d have to change the SwiftUI code for the form. Again, not onerous, but tedious. For one, I’d have to dig through all the files in Xcode. And what about backwards compatibility?
I came up with what I feel is a cleaner and better solution. Here are the broad strokes. I store all of my project data in a directory FileWrapper. Along with the actual data, I store metadata for each of the forms in the FileWrapper in JSON. Why? I’m not locked in to using Xcode should I wish to, for example, write a version of my writing app in Python.
Why store it in the package and not in the app bundle? The metadata flows with the project data. If I change them, the app will still be able to decode and use the data and metadata through versioning. If I change the data, all I have to do is change the JSON metadata file.
So how does it work?
In practice, I:
1) load the data into my model. Then, when I display a form, I
2) pull the JSON for that form from the FileWrapper,
3) decode JSON into a Swift dictionary,
4) map the data from my model to the dictionary, and
5) display the form using a custom binding
I don’t enjoy getting fancy, so there are no generics or code that requires an advanced degree.
Using this method, I can create any SwiftUI form I need.
In the next few posts, I’ll show the code and walk through the process.
Shifting Gears
I like to shift gears. I have lots of ideas and like to try them out. I’ve shifted gears, yet again. Having taken the first half of the year away from Xcode to let my mind and thoughts get away from having to play catchup because of everything new in WWDC, and having watched the sessions I’m interested in, I’m back in Xcode-land.
It’s still a monolithic program with new and interesting bugs, and some old ones. I won’t dwell on that. What I want to touch on is the 5th rewrite of my writing app, Auteureist. Having taken time away from Swift/SwiftUI to work on Python and The Continuum has given me a fresh outlook on what I want in a writing app. Auteureist has been a highly opinionated app, my opinion. I wrote it to work the way I write. As Swift and the platforms have evolved, so has how I write. That’s an aside. The point is, I’m rewriting Auteureist yet again.
I’m going for simplicity of design and UI while still keeping all the functionality. I’ve reworked how the writing projects are saved and organized. I’ve made the UI more “standard” and easier to use. The code base is simpler, and there is less of it. I’ve gotten a better handle on Swift and my code is more efficient. (I still hate the syntax, and don’t get me started on the new macros component.).
I’m currently working on the metadata editors. Before SwiftUI Forms, I designed my own. At some point, I created forms that “built themselves” given a JSON structure. Right now, I’m reworking the JSON structure, simplifying it and incorporating it into a SwiftUI Form. My proof-of-concept works. I read a JSON file from my package, convert it to a dictionary in Swift (easy-peasy), and then pass the dictionary to a SwiftUI View. This lays out the form and its components. So instead of having umpteen SwiftUI Views, I have one view that can theoretically generate any form given a JSON dictionary. This also lets me change any stored data without having to update SwiftUI code, by updating the JSON file. It works. It's a nice abstraction.
That’s the good news. The bad news is,. inspector which I used to display the form, works inconsistently between the various Apple platforms. This isn’t surprising, since. inspector is a new feature.
On the Mac it works as expected.
On the iPad isn’t detecting a tag I’m using in a switch statement, and the code falls through into the default case—but only if I use. inspector.
On the iPad mini. The. inspector is broken. It flies out into the middle of the screen, and only partially hides.
Same code. Three different results.
Oh well. Whatever the problem may be, I’m on the right track, and it will eventually be fixed.
Micro Projects Update
I’m making progress on my MicroProjects project. I’ve gone through several iterations of the core UI and am getting near something I like. I’m also working on the web and Swift/SwiftUI versions in parallel, along with my TinyDB wrapper which this uses. I’m trying to keep the UI consistent between the various platforms, but know that won’t be possible. I’m still far from done, and I’m sure the UI will change several times until I land on something that I want to use. I’m close.
This is the web Flask version.
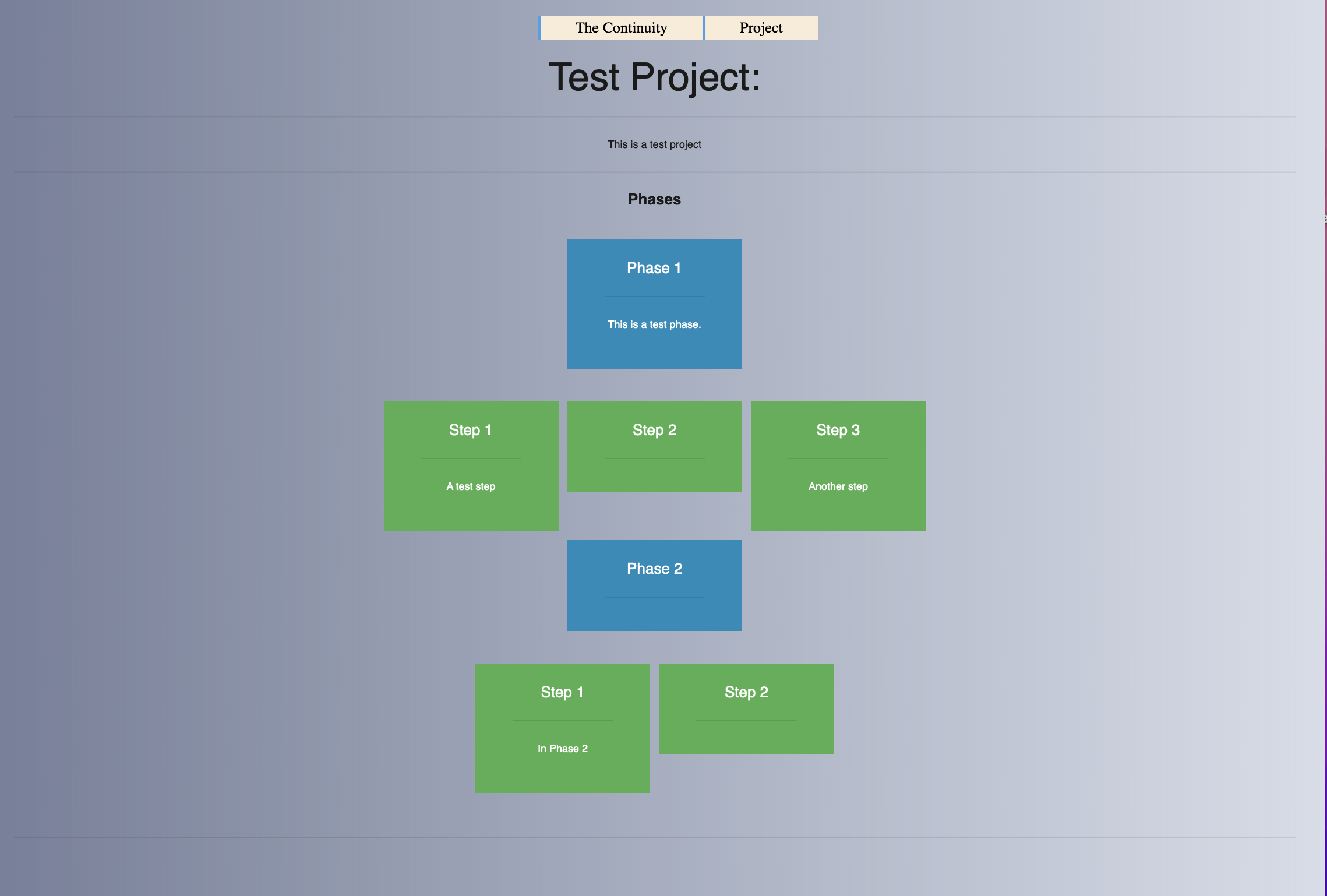
Here is the iOS version.
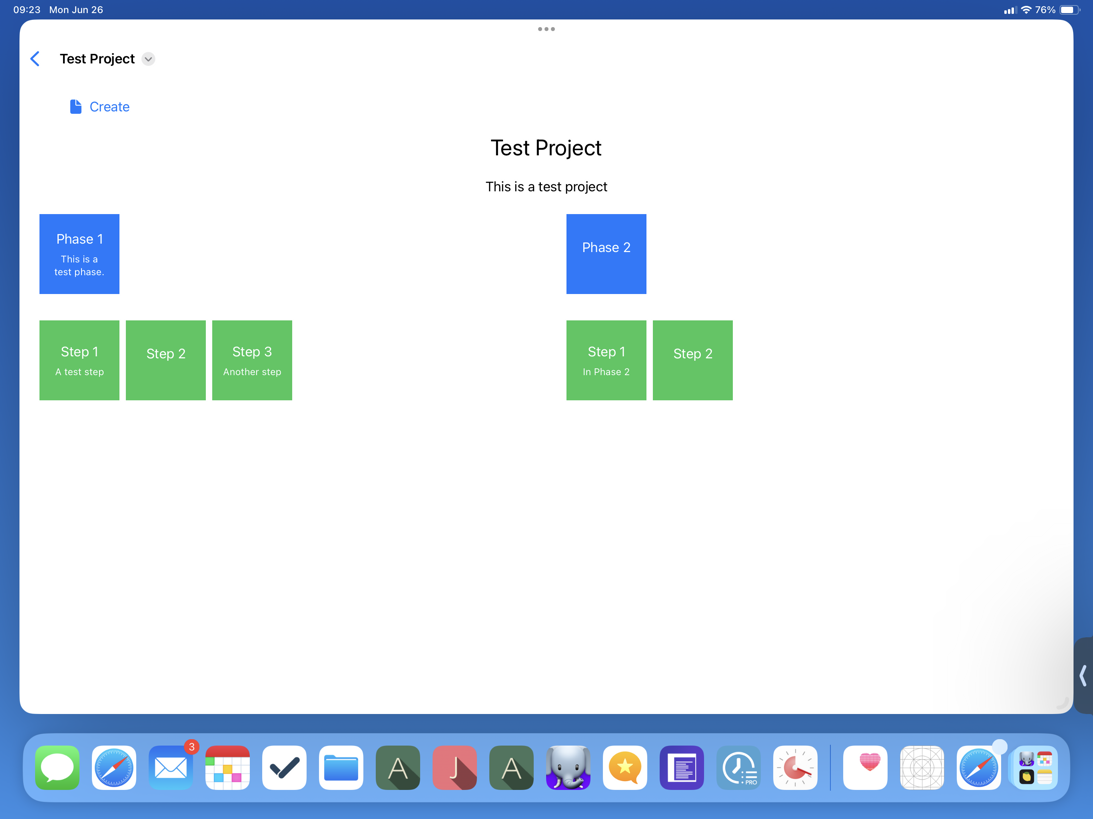
And the Mac version.
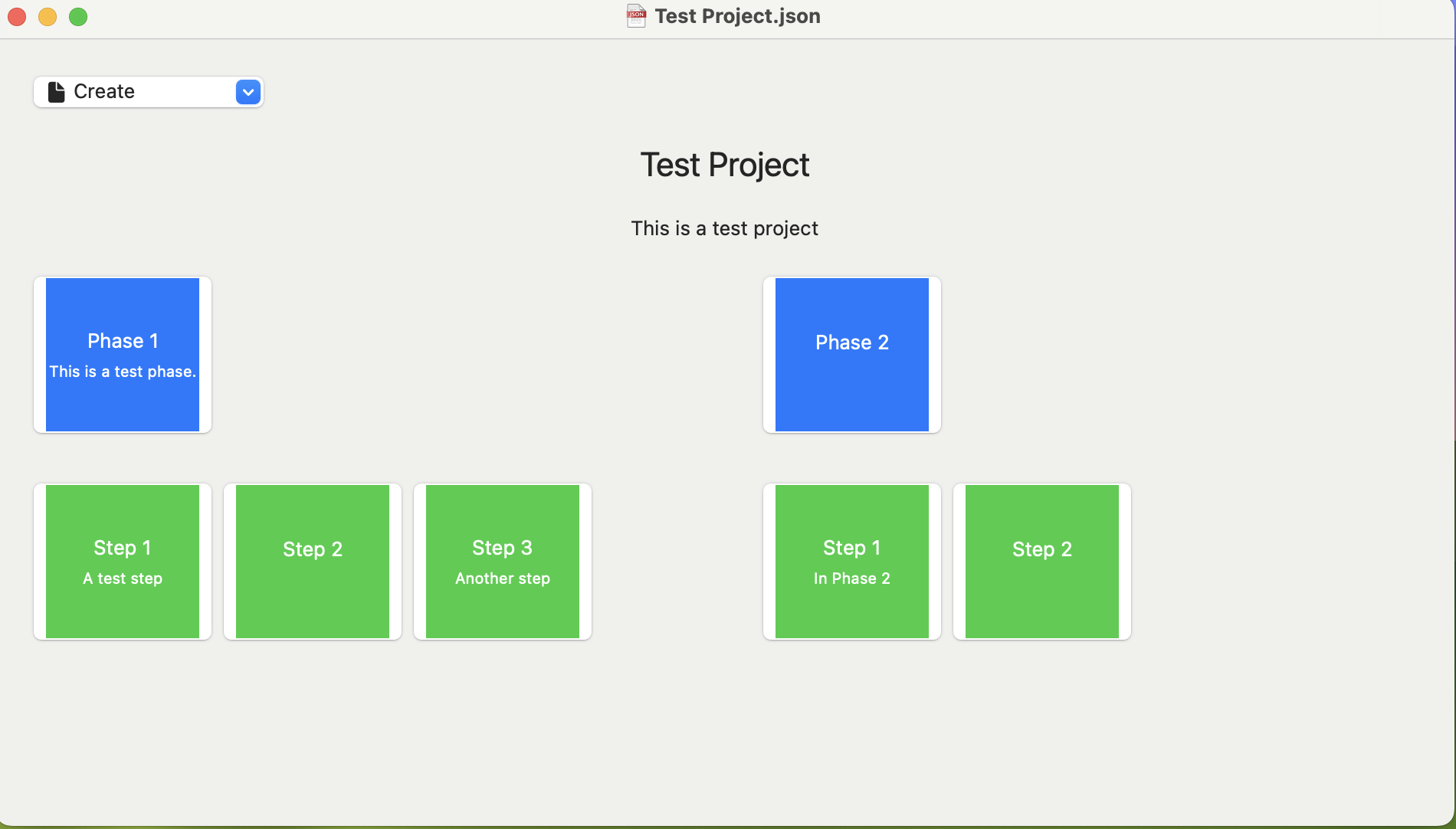
I discovered there is a problem with SwiftUI buttons on the Mac. Note the white vertical edges. I can’t seem to eliminate them, and they only occur on the Mac.
Swift TinyDB Library Progress and the New Xcode
I’ve begun work on the Swift TinyDB library. It’s a part-time project so I’m not making a lot of progress, but I’ve made some. I’ve gotten my library to create a database and read my reference databases. This was simple to do since TinyDB databases are JSON. The hard part is yet to come. I’ll have to mimic the TinyDB calls in Swift and provide the appropriate functionality and metadata of the database.
Being a library, there is no UI, but I’m working on my MicroProjects app in parallel since it uses the library. In addition, to standard XCT tests, the app is a nice test-bed.
I’m using the beta version of Xcode that was released at WWDC. Although, it’s still a monolith, Xcode seems better. The code completion is nice, and I’m really liking the new SwiftDocC documentation preview. I’ve never used DocC, but this seemed like a good year to start using it. It’s well implemented and works. One feature I’d love to have is the ability to build the documentation as an ePub so you could SwiftDocC to write standalone manuals. I looked at the output documentation and it’s just a folder/FileWrapper with a lot of HTML, CSS, and JSON files. So writing a utility to convert it to an ePub is doable. I just don’t have the time. If you’re up to it, go for it.
The improvements in Xcode’s git integration are welcome, but it’s still not as full-featured as Tower. For quick commits and such, it’s good enough.
One thing I don’t like about this version of Xcode is the auto-indentation, especially when creating documentation. It seems unintuitive, and seems to mix tabs with spaces. I haven’t researched it enough, I guess.
I’ve only scratched the surface of this Xcode beta. I still hate that it’s a single conglomeration of a program. I don’t really understand why Apple doesn’t allow plug-ins. I’ve said this before. Xcode should be a product suite.
At some level, it probably has to do with security or the integrity of the application. But Apple is a smart company. They could solve whatever problems they may see.
TinyDB
I mentioned that I am using TinyDB in my Flask website. TinyDB is a NoSQL database similar to MongoDB and CouchDB. I’ve been a big fan of MongoDB from day one, but because it no longer runs on the latest RaspberryPi OS, I looked for alternatives. I’m using CouchDB for other projects, but I prefer TinyDB because it doesn’t require a server and produces stand-alone databases in JSON.
That means, I can use TinyDB across platforms, and where the database needs to be portable, such as on iOS. Unfortunately, there is no implementation of a TinyDB library, so I’m writing my own. I’m going to keep the library call syntax close to the Python implementation of TinyDB’s simplifying porting code to iOS.
What of SwiftData, which was announced at WWDC this year, I hear you ask? Well, I haven’t gone down that rabbit hole yet. I’ve seen the high-level presentations, and it looks interesting, but from what I can tell, SwiftData is another relational model layer, better suited to CoreData and SQL databases. Now, I have nothing against SQL. In fact, I spent “many-a-year” in it and I’m expert in it both from a design and access standpoint. But document-based storage makes more sense for what I’m trying to build. SwiftData may link to a NoSQL engine, but I haven’t looked into that. That’s the reason for TinyDB.
Today, I started an XCode project, with the latest beta. I’m working on being able to read a TinyDB database since I have some reference examples I’ve created on other platforms. Although TinyDB is JSON, it has some interesting concepts. It has the concept of tables. A TinyDB database can store related documents in these tables—all in a single file. It’s like SQLite, but with JSON documents. TinyDB allows you to create custom middleware and storage engines. The example they provide is using YAML instead of JSON. I won’t be writing my own middleware or storage solutions, since I don’t need that capability for what I’m doing. I just want to read an existing database first. Then I’ll worry about manipulating it.
Depending on how things go, and how clean the code is, I may release it into the wild. We’ll see. If you want to learn more about TinyDB, check out their documentation site. https://tinydb.readthedocs.io/
The Continuity
I’ve taken time away from Swift/SwiftUI in the months leading up to WWDC. I’ve found I usually have a lot of code to redo once WWDC’s announcements become public. This year, I decided to not code Apple stuff between January and WWDC.
It’s not that I haven’t been coding. I took the break to learn something new. I dove into learning Flask, a micro web framework written in and for Python. I’ve been having fun, which I rarely have when fighting XCode.
it’s nice to use a mature and stable programming language where I don't feel like I’m trying to hit a moving target.
When I began my Flask journey, I wasn’t sure what I wanted to make, so I started with a basic website. Flask might be a micro-framework, but that doesn’t mean it’s not full-featured.
Once I understood the basics of Flask, I began thinking a database would be nice. I usually use SQLite for my own projects and that’s what I used with Flask and a plug-in known as SQLAlchemy.
I then thought a journaling and life stream app of the sort I have on iOS would be worth building in Flask. Then, I ran into a problem.
The idea floodgates opened. I added functionality and more functionality. Now I have something pretty useful, and I think, amazing with the little time I’ve had to spend.
I called my project “The Continuity” which refers to the continuity of my thoughts and actions. A life stream. The site lets me pull sites and post information to:
Social networks:
- Micro.blog
- Mastodon
- Twitter (until the API dies)
- OMG.lol
Other stuff:
- Weather
- Stock market
- Goodreads
- Cheerlights
I’ve also linked my external and internal sites. I installed Codeserver (the server version of VS Code) which I now use to develop all of this. Then I discovered the concept of Micro Journals and added functionality for that.
I’ve always wanted a way to track my own projects the way I work, and am working on what I call MicroProjects.
The Continuity has expanded beyond my initial desire to learn Flask. I’m still learning, for example, how to do forms and secure login.
I’ve also switched most of my database from SQLite to CouchDB, which I had to use because MongoDB wouldn’t run on the RaspberryPi that The Continuity uses. That led me down a No-SQL rabbit hole and I stumbled on a database called TinyDB.
TinyDB is a small standalone No-SQL implementation. It’s perfect for cross-platform use. Which led me to decide I should rewrite my iOS journaling app to use it. But there is no library for TinyDB on iOS/Mac. I’ve started writing one.
Being able to use the same files from the web and iOS and Mac is great, but I had to figure out how to get an iPhone to get to a RaspberryPi DB and vice versa. I’ve had to deal with Swift and its network and security hoops before. I didn’t want to jump through them.
So I figured out how to synchronize iCloud files to the RaspberryPi. Now I’ll be able to work on either platform without having to deal with network protocols and security. A big win for me.
All of this progress in probably less than a month of work. I started out wanting to learn Flask. I’ve learned so much more along the way.
Now that WWDC is winding down, I’ll switch gears a bit and get back to some iOS/Mac development. I do want to redo my journaling app, and maybe my writing app (yet again). Being able to use TinyDB as the data store will simplify the code and functionality a great deal.
I also want to create a MicroJournals and MicroProjects app for iOS/Mac.
No, I probably won’t release them, since I don’t want to deal with managing users or the codebase. But I think I’ll start posting about various bits of The Continuity, so you can see what it’s really about and to help me focus.
Let me finish up by saying, learning something new is never a waste of time. You never know what you’ll discover about the topic or what you’re capable of.
WWDC 2023 Thoughts
This is Apple’s WWDC week. Apple announced the usual OS upgrades and new features, plus some new things. Most of the new stuff premiered at the keynote I wasn’t interested in. Things such as new stickers, FaceTime improvements, and even though are cool from a developer standpoint, it’s stuff I don’t want or need.
I was looking forward to the new journal app. Honestly, I was expecting more, and was disappointed. I was hoping to see more integration and automation—something similar to the Day One app. Unless there is more than what was revealed in the keynote, it’s very manual and rudimentary. It’s more of a “highlights” and some basic prompting style of journal, rather than a life stream. I’ll stick with my app, unless developer details change my mind.
Of more interest are the new Health capabilities. Cycling is now more full-featured. Including mental health is a big plus. Both are interesting from a user and developer standpoint.
The Apple Watch got some love, as I hoped. But again, it looks more like a minor facelift. I’m still hoping the developer tools have been upped-a-notch.
iOS and iPadOS got some features I’ll make use of, I’m sure. Live widgets are an improvement.
MacOS…it got a new name—Sonoma. I really remember nothing from that part of the keynote apart from widgets. So, I’m assuming there isn’t much new chrome. Apple usually makes a big deal about the new look each year. There wasn’t really much in this part of the presentation. Again, I’m hoping there is more under-the-hood.
New hardware. A new M2 MacBook Air. That’s something I want. I’ll stick with the 13” version. I can trade my existing Air for a nice reduced price.
Other new M2 Macs are cool, and the Pro is a beast, but it’s not something I need, or really want.
The big news was Apple VisionPro, the new AVR set. I found it interesting that Apple is marketing this as a new type of computer, rather than a gaming/lifestyle headset. I think the price has something to do with that, but more on that later. The thought and technology that went into the design are astounding. I won’t go into it all, but a couple of things jumped out at me. First, unlike Sony’s new PSVR2, you wear nothing on your hands. VisionPro senses your hand gestures with nothing, which makes using it more intuitive. Second, you can use it with a physical keyboard and trackpad. I hadn’t considered this as an option, but it makes perfect sense and Apple made the right decision. Third, the headset has a 3D camera (with video) built-in. That’s impressive. Finally, the faceplate is actually a 3D screen that displays your eyes and other things to observers. All this requires a new 3D OS. I am also surprised the battery pack isn’t very large. I was expecting it to be much bulkier. Unfortunately, the almost $4K price tag is out of my range, and I think most one-man developer shops. Oh well. It is, however, cool tech. One other thing I found interesting. The product is called Apple VisionPro, which to me, implies there must be an Apple Vision, or even an Apple VisionLite for us unwealthy mortals.
It was a mixed keynote for me. There were a few interesting things I’ll make use of. I’m looking forward to seeing some sessions and pulling down the latest software.
WWDC 2023 - What I Would Like to See
With WWDC 2023 coming next week, I thought it would be a good time to bring back this blog. As with all my blogs, I focus on keeping my ideas and progress straight. It’s never about followers or subscribers. As always, I hope, however, that you may find something interesting here.
What I Want (In my personal priority order)
- A journaling app that puts my own and Day One to shame. Apple has the data to make a pretty great life-stream app. It all comes down to how much they think it’s worth having, which will determine how great the UI is. I would like to stop working on my own journaling app and focus on my writing app and other projects. Apple could make me happy. I’ll see.
- A better Books app and an API/framework for developers. I love the Books app, but the UI and the inability to better organize books is a pain.
- More SwiftUI “widgets”.
- A simpler-to-use Combine, both conceptually and syntactically. I avoid Combine because I don’t enjoy jumping through the hoops it places.
- A faster-to-install and slimmer XCode. Installing XCode takes too long, even with the more powerful CPUs. I’d also love an XCode “suite”, instead of having a single monolithic app. This would also make the Playgrounds app more powerful if bits of the suite could be used by Playgrounds on iPad.
- Speaking of which, I want app development in the Playgrounds app to be a bit more powerful. It’s pretty cool the way it is. I’d like to have it split from the training and learning aspect of the app, into its own app.
- A simpler CoreData would be nice that would allow me to use whatever database I want to. Nowadays, I’m using CouchDB and tinyDB (along with SQLite). I remember the pain CoreData instilled on me in its early days—great idea, poorly executed.
- I’d like to have XCode opened up to support other languages. Nowadays, I’m doing a ton of Python and DB stuff. This would be possible if Apple split out XCode into a suite, as I mentioned above, and you could write language plugins for the code editor portion. I won’t hold my breath. Apple would like us all to use Swift/SwiftUI—which is great, but limiting in so many ways.
- Hardware? It seems everyone and her dog are expecting Apple’s AVR headset with drooling breath. Given the rumored price tag, I’ll pass. Also, it’s not something I need. I’m more interested in a new iPadMini. I think I’ll pass on this year’s iPhone upgrade. First, I got one last year, and second, the increased size rumors are putting me off. I’d like to see Apple buy out Oura, the makers of Oura ring. The Apple Watch and Oura ring are very complimentary when it comes to health tracking. I also find I prefer sleeping wearing the ring instead of the watch. The ring’s battery also outlasts the watch’s by several days.
- As far as the watch goes, I doubt we’ll see a new one announced at WWDC. But if they do, I’d love to see some new sensors integrated with API/frameworks. I’d like to see a lot more developer love given to the Apple Watch.
That’s all I’m looking for. I’m sure Apple will have lots of new developer tools/goodies I’ll have to dive into and play catch-up. Oh, I’d love to see Apple introduce a release cycle of once every six months instead of every week or two. But that’s me.
‘til next time.
Welcome
This is a blog where I will post my thoughts and progress on developing stuff. This will be a "sometimes" blog.