Nesting Structs
07/26/21 08:30 Filed in: GO
Last time I learned about how to use pointers in GO to pass strutcts to functions. Today, I figure out if there is anything I should know about nesting a struct within another struct. Read More…
Structs and Pointers
07/12/21 08:35 Filed in: GO
Last time I mentioned that GO passes parameters by value, so modifying a struct within a function takes extra work. If my structs are large, there may be memory pressure as a result of creating copies in functions. That's where passing a pointer to a struct can help. That's what I tackle this time. Read More…
Structs in GO
06/28/21 08:27 Filed in: GO
I've learned about maps and slices in GO. However, they can only hold items of uniform types. Today, I dive into learning about structs which can hold a combination of different types.
Structs are a common data structure in many programming languages and are useful for managing real-world records and related information of different types. The syntax is also similar to that of other languages.
var name struct {
variable type
variable type
variable type
…
}
You can have any number of variables in a struct. Like other things in GO, the default values are the appropriate zero values for the data types. Here is an example:
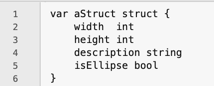
In order to set values you use the dot operator.
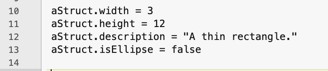
This is similar to how other programming languages do it, as well.
If I need more than one record based on aStruct, I can either repeat the definition of the struct, or I can create a new type based on it. I can then declare variables based on the type. So, declare once, use often. Here is the syntax for a defined type based on a struct.
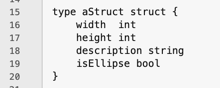
This looks like the same code. There is one major difference: the type keyword replaces the var keyword. Once defined this way, I can declare variables based on the type:
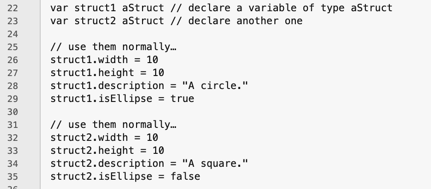
Being a type, I can pass a struct into a function as an argument.
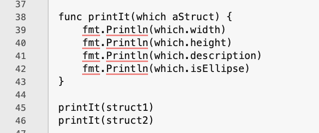
One thing I need to remember is that GO passes parameters by value, so modifying a struct within a function takes extra work. It's better to treat GO functions as pure functions and return a modified copy.
There is an issue. If my structs are large, there may be memory pressure as a result of creating copies in functions. That's where passing a pointer to to a struct can help.
I'll tackle that next time.
Structs
Structs are a common data structure in many programming languages and are useful for managing real-world records and related information of different types. The syntax is also similar to that of other languages.
var name struct {
variable type
variable type
variable type
…
}
You can have any number of variables in a struct. Like other things in GO, the default values are the appropriate zero values for the data types. Here is an example:
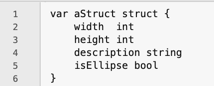
Assigning Values to a Struct
In order to set values you use the dot operator.
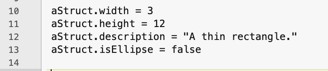
This is similar to how other programming languages do it, as well.
Type Definition
If I need more than one record based on aStruct, I can either repeat the definition of the struct, or I can create a new type based on it. I can then declare variables based on the type. So, declare once, use often. Here is the syntax for a defined type based on a struct.
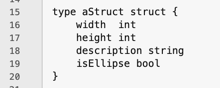
This looks like the same code. There is one major difference: the type keyword replaces the var keyword. Once defined this way, I can declare variables based on the type:
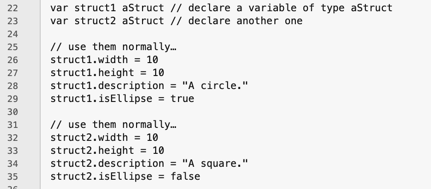
Structs and Functions
Being a type, I can pass a struct into a function as an argument.
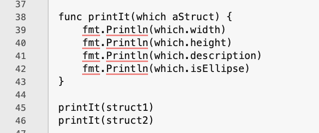
One thing I need to remember is that GO passes parameters by value, so modifying a struct within a function takes extra work. It's better to treat GO functions as pure functions and return a modified copy.
There is an issue. If my structs are large, there may be memory pressure as a result of creating copies in functions. That's where passing a pointer to to a struct can help.
I'll tackle that next time.
Iterating Maps
06/14/21 08:42 Filed in: GO
Last time I learned about maps in GO and how to create, change, and delete them. Today, I go over iterating over a map. Read More…
Variadic Functions
05/17/21 07:47 Filed in: GO
Command-Line Arguments
04/26/21 07:43 Filed in: GO
Today I learn about processing command-line arguments in GO. Read More…
Slices
04/12/21 08:18 Filed in: GO
Today I dive into something called a "slice" in GO. A slice is a collection that can change size to accommodate a changing number of elements. Arrays in GO are fixed to the size they are declared.
Read More…
Read More…
Reading Files Into Arrays
04/05/21 08:22 Filed in: GO
Last time I learned how to read a text file. I continue my investigation of reading text files by loading a file full of numbers into an array. Along the way I learn about converting numbers. Read More…
Reading Text Files
03/29/21 08:50 Filed in: GO
I read a lot of text, and other, filed. Today, I want to tackle a common function, so to speak. How to read a text file. Read More…
Arrays In GO
03/15/21 09:31 Filed in: GO
I'm done with GO packages for now. I want to get back to the core language. Today I learn about arrays. Read More…
More on Packages
03/08/21 08:15 Filed in: GO
Last time I learned about writing and importing my own packages. This time, I want to learn about installing and publishing packages.
Read More…
Read More…
Packages
03/01/21 08:32 Filed in: GO
In one of the first posts I wondered why fmt.Println() used a lowercase fmt and an uppercase Println. In this post I dive into GO packages which answer the question. Read More…
The Point of Pointers
02/22/21 08:07 Filed in: GO
Last time I learned about GO functions and parameters. Today, I want to learn about addresses and pointers in GO.
Read More…
Read More…
More On Printing & Functions
02/15/21 08:21 Filed in: GO
Today I'm going to cover formatting output in GO, as well as functions. Read More…
Things To Remember & For Loops
02/08/21 08:34 Filed in: GO
Going through the GO docs and a couple of my books, I've come across a couple things I need to remember when dealing with the scope of variables.
Read More…
Read More…
More Useful Functions
02/01/21 08:09 Filed in: GO
Last time I learned about using the time package, substring replacement, and getting user input. I learned functions can return more than one value, including an error. Today, I look into handling returned errors and the if statement. I'll also tackle casting strings to numbers. Read More…
Useful Functions
01/25/21 08:23 Filed in: GO
Today, I want to go over a few useful functions.
Read More…
Read More…
Installation & Basic Commands
01/18/21 08:28 Filed in: GO
Last time, I looked at variables and variable naming. This time I'm gong to install GO and then look at compiling and running programs.
Read More…
Read More…
Variables and Variable Names
01/11/21 08:36 Filed in: GO
In this post I'm going to focus on more basics of the GO language, data types and variables. Read More…