Handling Collisions
03/31/21 08:22 Filed in: PICO-8
Last time, I introduced a function to detect collisions, I got Ferb jumping over rocks as objects, and have an asteroid falling from the sky. Today, I want to do something whenever Ferb hits a rock or is hit by the asteroid. We're on our way to a simple game.
You may be wondering why I'm not going to check for an asteroid hitting the ground. I've already handled that by making the asteroid disappear when it hits. I think I want an explosion at that point, but I'll deal with that in the future. For now, I want to handle the other collisions.
Just a note: After the last post, I did some refactoring and converted Ferb into an object to be more consistent with rocks and meteors. The collision function I introduced last time also requires objects. You can get the full source code in the cart at the end of this post.
Add Hit Boxes
As a reminder, my collision detection function uses hit boxes around the sprite. That means, I need to add a hit boxes around Ferb, the rocks, and the asteroid. First, I want to add it whenever I initialize Ferb.
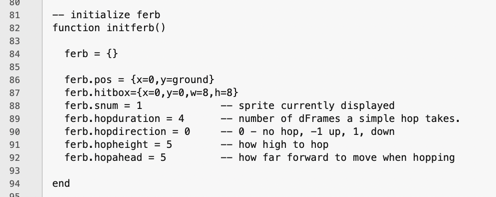
Line 87 is all we need. The thing to remember is the collision function automatically adjusts the position of the hit box to match the sprite, so the x, y of the hit box are always going to be 0. That is, the hit box will automatically track the position of the sprite.
Now for the meteor, line 228 is where I initialize the meteor.
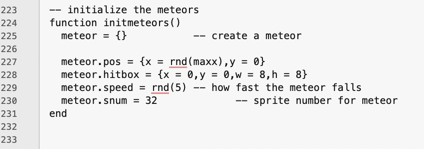
And now, the rocks. You see the pattern. Line 265 adds the hit box.
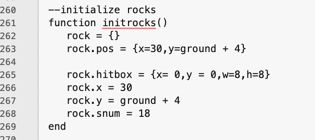
Checking For Collisions
Now, all I should have to do is check for Ferb running into a rock or a meteor.
One thing to remember is that I can't do any drawing outside the _draw() function. That means I need a way to tell the _draw function that Ferb hit something. The easiest way to do this is with a flag. I need one for when Ferb hits a rock, and one when Ferb hits a meteor. I don't care which rock or meteor, so I only need one flag.
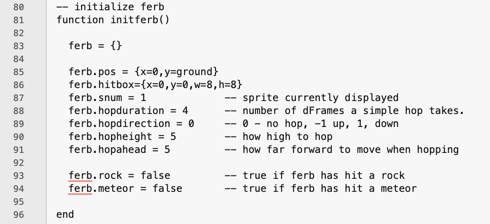
First, I'll use my collision function and pass in the Ferb and Rock objects and set the flag if they collide.
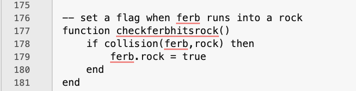
And a similar function for checking when ferb hits a meteor.
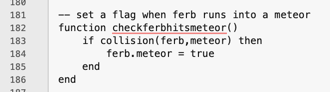
I'll call these whenever I update Ferb's position.
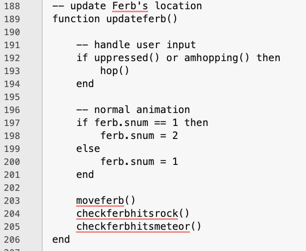
Finally, I need to draw something whenever threre's a collision. I'll just print a string.
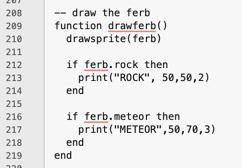
I'll want to do something more "game-like", but I'm just testing now. That should be enough. Let's run it.
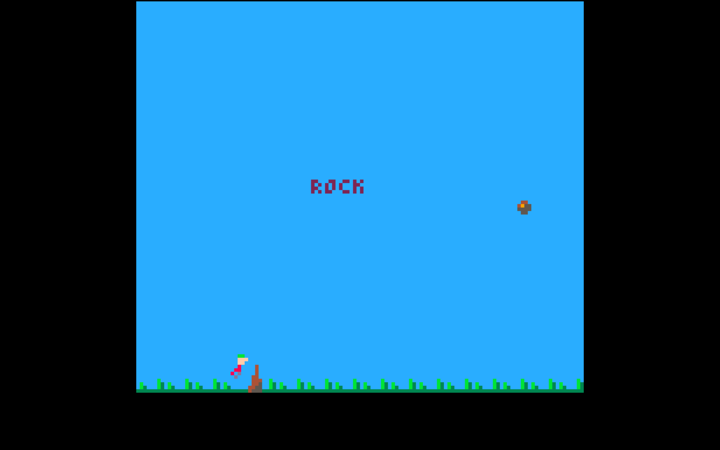
Ok, it works, but there is a new problem. The hit box is too big (or Ferb can't jump well enough). I'll adjust the hit box to closer hug the rock and meteor (make it smaller). After playing around a bit, I settled on:
- ferb.hitbox = {x = 2,y = 0,w = 6,h = 6}
- meteor.hitbox = {x = 0,y = 0,w = 8,h = 8}
- rock.hitbox = {x = 4,y = 0,w = 4,h = 4}
With the Ferb, and rock, not only did I make the hotbox smaller, I adjusted the x coordinate to be tighter to the actual body (smaller border). Running this produces a decent result.
At this point, I have the start of a game. Next time, I'll do some collision animations.
As always, here's the cart and source code.
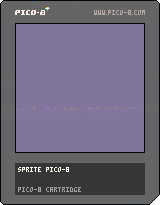